Default Nodes
@bluepjs coming with default set of Nodes. New Nodes can be added be Developers
Note
Current list of Nodes is small cuz was developed “on demand” and doesn’t contains all basic operations with different types.
New Nodes will be updated in next versions.
Booleans
And (A && B)
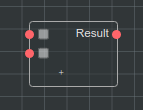
Modifier node with multiple inputs.
Set output “Result” as logical AND of inputs.
Or (A || B)
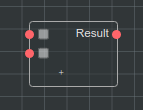
Modifier node with multiple inputs.
Set output “Result” as logical OR of inputs.
Not (!A)
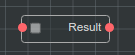
Modifier node.
Set output “Result” as logical NOT of input.
Equal (A === B)
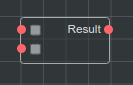
Modifier node.
Set output “Result” as A === B
Numbers
Equal (A === B)
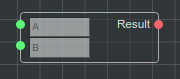
Modifier node.
Set output “Result” as A === B
Is even
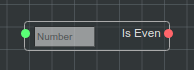
Modifier node.
Set output “Result” to true
if input number is even.
Greater or equal then
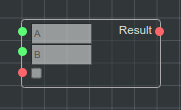
Modifier node.
Set output “Result” to true
if input A is greater then input B (or equal if boolean input is true
).
To String
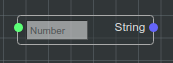
Modifier node.
Stringify input number.
Plus (A + B)
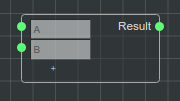
Modifier node with multiple inputs.
Set output “Result” as summ of inputs
Strings
Append (A + B)
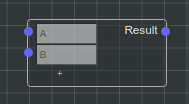
Modifier node with multiple inputs.
Set output “Result” as united inputs.
Datetime
Note
@bluepjs uses dayjs
(website) library to manage date/time/datetime.
Create
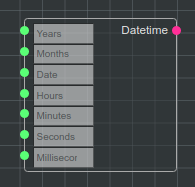
Modifier node.
Creates basic/datetime
object using numbers input.
Unwrap
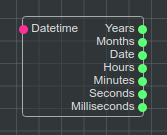
Modifier node.
Unwrap basic/datetime
object into numbers.
To String
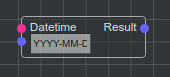
Modifier node.
Formats basic/datetime
into string by format template.
Note
Check dayjs format docs.
Now
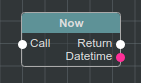
Executable node.
Created basic/datetime
output with current datetime.
Undefined
Is undefined
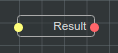
Modifier node.
Set “Result” output to true
if input is undefined.
Note
javascript: typeof input === 'undefined'
Branches and execution flow
For
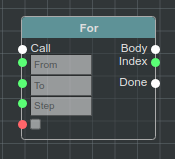
Executable node.
For loop to iterate by ‘from-to-step’
Each
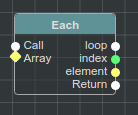
Executable node.
Each loop to iterate arrays.
If
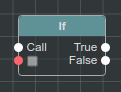
Executable node.
If/else node.
Switch .. case
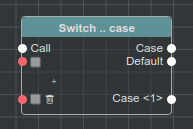
Executable node with multiple inputs/outputs.
Switch .. case node.
Call
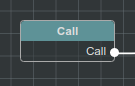
Executable node.
Autogenerated by IDE. Can’t be added/removed manualy.
Return
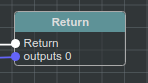
Executable node.
Can be added for Functions with outputs.
Wait
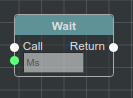
Executable node.
Pause execution flow for ms input.
Function
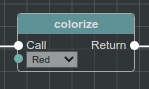
Dynamic executable node.
Autogenerated by IDE nodes for each available Function.
Note
Colorize function described in extended integration example
Others
Console log
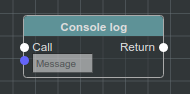
Executable node.
Prints message to Vm console.
Enums
Enums nodes are autogenerated by IDE for each enum
Equal
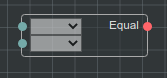
Modifier node.
Check if inputs are same.
To String
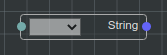
Modifier node.
Stringify input enum value.
Structs
Structs nodes are autogenerated by IDE for each struct.
Note
Struct on images described in extended integration example.
Pack
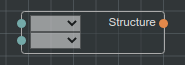
Modifier node.
Creates struct by fields.
Unpack
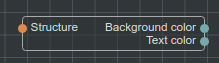
Modifier node.
Unpack struct by fields.
To Object
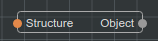
Modifier node.
Convert type from struct type to basic/object
(javascript object)
From Object
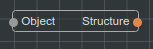
Modifier node.
Convert type from basic/object
(javascript object) to struct type.
Function inputs/outputs/variables, class properties
For any Function (simple function, event function, class constructor or method) there are autogenerated by IDE getters and setters Nodes.
Generating depends on variable context and access for class properties.
Get
Get Function variable:
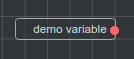
Get Class property:
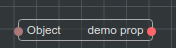
Getter node.
Get required variable/property. For Classes takes object of class to modify.
Set
Set Function variable:
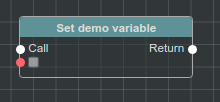
Set Class property:
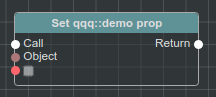
Executable node.
Set required variable/property. For Classes takes object of class to modify.
Classes
Classes Nodes are autogenerated by Classes and Actors.
This

Getter node.
Exists only in Class methods and constructor blueprints. Returns “this” pointer of object.
Cast to
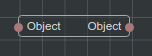
Modifier node.
Exists only when some Classes Inheritance is found. Convers object type from parent type to child type.
New
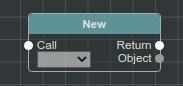
Executalbe dynamic node.
Exists only when some Classes exists. Creates new object of class. Node inputs are autogenerated by selected constructor inputs.
Constructor
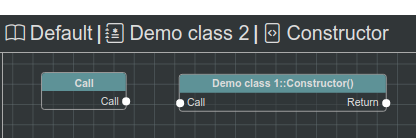
Executalbe dynamic node.
Exists only when some Classes Inheritance exists in child classes constructors. Allows call (or not) parent class constructors.
Note
Example image demostractes “Demo class 2” constructor. “Demo class 2” defined as extends from “Demo Class 1”.
Method
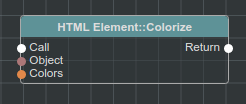
Executalbe dynamic node.
Autogenerated by IDE for Classes/Actors methods with accesibility checking (public/protected/private/…). Takes object to use as input.
Note
Colorize function described in extended integration example